OpenAI recently introduced Codex, a powerful AI agent designed to streamline software engineering tasks. Built on the Codex-1 model, this cloud-based tool handles code review, refactoring, error handling, and test generation—allowing developers to focus on innovation and architecture.
Codex differentiates itself by offering specialized assistance tailored specifically to software engineering, effectively bridging the gap between AI assistance and human expertise.
In this article, we'll explore Codex's capabilities and demonstrate how experienced developers can benefit from this AI teammate.
What is OpenAI Codex
Codex is an AI assistant built on OpenAI's Codex-1 model, adapted from the o3 reasoning model. It is designed to handle repetitive engineering tasks, enabling teams to prioritize high-level design and innovation.
Imagine switching your streaming platform from TypeScript to Golang to improve runtime efficiency. Codex simplifies this migration, managing codebase refactoring efficiently in the background.
Codex runs in a cloud-based environment, enabling parallel execution of tasks based on natural language prompts and existing codebases. OpenAI trained Codex using reinforcement learning on real-world coding tasks, ensuring it closely mirrors human coding styles and preferences.
Using Codex
The Codex agent is currently available for users on OpenAI's Pro subscription, which gives you access to the Codex UI and its CLI. You interact with it much like you would a pair programmer — using natural language to guide its actions within your codebase.
Core Capacities
One of the most powerful features of Codex is its tight integration with GitHub, where it mimics real-world developer behavior. It doesn't just analyze code — it can review pull requests, write commit messages, summarize diffs, and even suggest or make safe changes inline.
For example, after a feature branch passes tests, Codex can:
- Review pull requests
- Write context-aware commit messages
- Summarize diffs
- Suggest or apply safe inline changes
- Automate merges based on CI conditions (with approval)
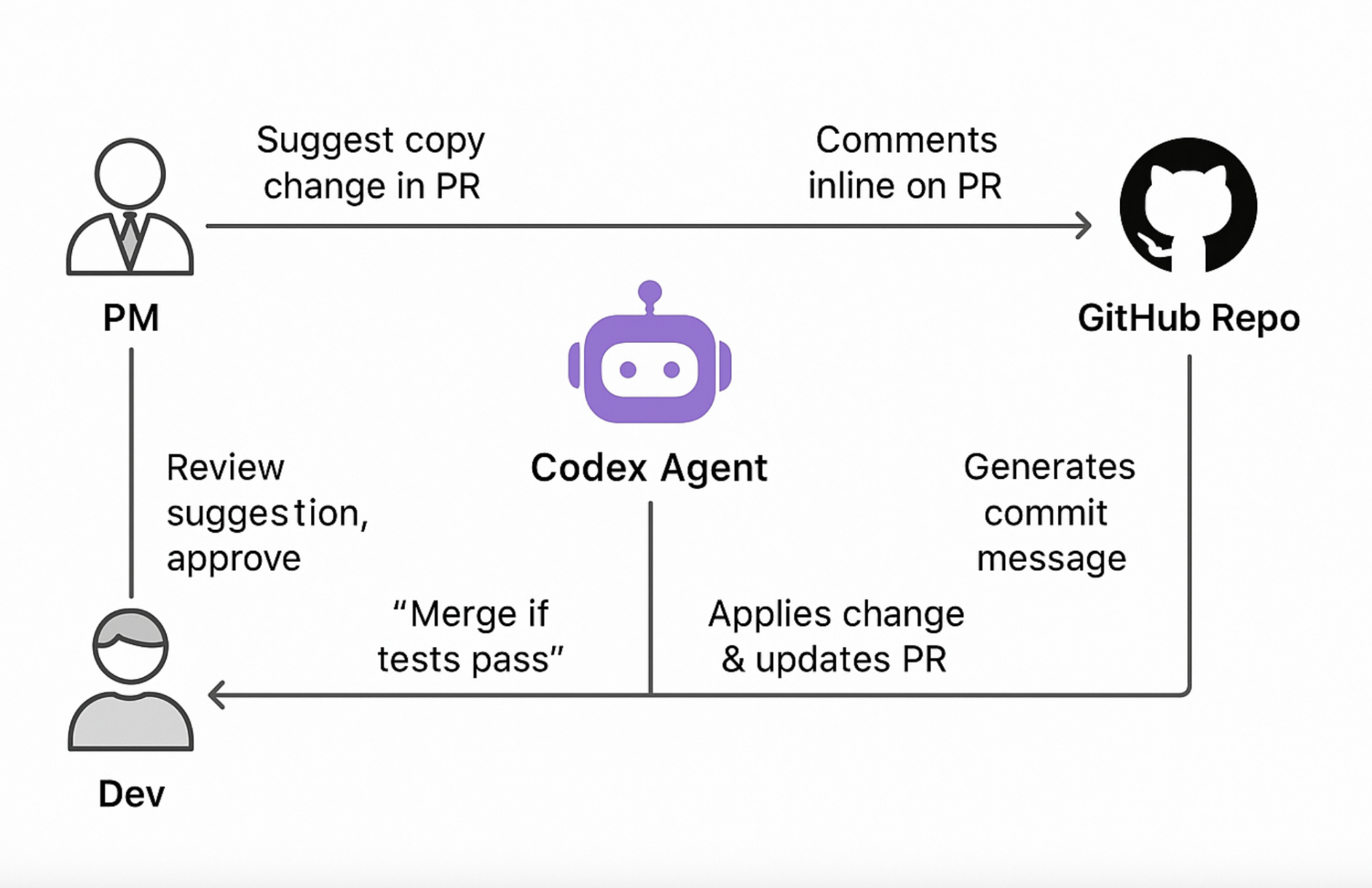
Non-Engineer Collaboration
- Suggest copy changes
- Adjust basic logic
- Provide direct implementation feedback
All of this without needing to spin up a local dev environment or schedule a sync. It opens the door for asynchronous, low-friction collaboration, especially for fast-moving product teams.
Codex is built to reduce the back-and-forth typically needed for small tweaks and feedback. Think of it as shortening the loop between idea and implementation — from a two-day Slack thread to a two-minute edit.
CLI Interaction
Developers can utilize the Codex CLI to:
- Generate and refactor code
- Test logic and debug issues
- Automate repetitive tasks
Real-World Demo: Refactoring Node-Todo App
To understand how OpenAI Codex performs beyond the demo reel, let's put it to the test with a real-world task: refactoring a simple node-todo app https://github.com/scotch-io/node-todo
, extending it with authentication, error handling, and improved readability.
We'll be using the node-todo open-source repo as our example case.
Refactoring node-todo with OpenAI Codex
First Codex Command
codex --auto-edit "Convert the app from callbacks to promises using async/await"
Codex combs through the codebase, identifies every callback‑based database call, and proposes async/await
replacements.
Below is a representative example for the GET /api/todos route. The original code looked like this—pretty minimal: no input validation, no structured error handling, no explicit status codes:
app.get('/api/todos', function (req, res) {
// Use mongoose to get all todos in the database
getTodos(res);
});
Codex's suggested patch
// AFTER (Codex‑generated)
app.get('/api/todos', async (req, res) => {
try {
const todos = await Todo.find();
res.status(200).json(todos);
} catch (err) {
res.status(500).json({ error: err.message });
}
});
What codex got right
- Context‑aware upgrade: It detected the callback pattern, swapped in
await Todo.find()
and wrapped the logic in atry/catch
block—no manual hints required. - Proper status codes: Successful responses now return 200 OK; errors return 500 Internal Server Error with a JSON payload.
- Production‑grade output: The patch is drop‑in ready and follows modern Express best practices.
- Time saved: Manual refactoring of every route (plus re‑testing) would easily take 5–10 minutes; Codex delivered working code in under 10 seconds.
Adding new features with Codex
Second Codex command
codex --auto-edit "Add a /completed route to get all completed todos"
The goal is to add a new route that returns only the completed tasks. Codex understood the data model (based on Todo.find()
calls earlier) and assumed a completed field existed on the schema.
Codex's Suggested Patch
Here's the code it generates:
app.get('/api/todos/completed', async (req, res) => {
try {
const completedTodos = await Todo.find({ completed: true });
res.json(completedTodos);
} catch (err) {
res.status(500).send(err.message);
}
});
What Codex Got Right
- Smart schema inference: Codex inferred the schema even though it wasn't explicitly defined in the command. It assumed a
completed: true
filter would be valid, which aligned with the rest of the code. - Proper placement: The route was inserted in
app/routes.js
right alongside existing endpoints, maintaining the structure. - Error handling included: It automatically wrapped the handler with try/catch, even though we didn't ask for it.
Time saved: About 5–10 minutes of manual coding and debugging was collapsed into a single command and instant patch.
Writing Tests with Codex
Third Codex Command
codex --auto-edit "Write a test using Mocha for the POST /api/todos endpoint"
The goal here is to automatically generate a unit or integration test for the /api/todos
endpoint in the app, using Mocha (a JavaScript test framework) along with Supertest (to simulate HTTP requests) and possibly Chai (for assertions).
Codex's Suggested Patch
Here's the code generated:
// *** Add File: test/todos.test.js
const request = require('supertest');
const chai = require('chai');
const app = require('../server');
const expect = chai.expect;
describe('POST /api/todos', function () {
it('should create a new todo and return an array of todos', function (done) {
const text = 'Test todo item';
request(app)
.post('/api/todos')
.send({ text })
.expect('Content-Type', /json/)
.expect(200)
.end(function (err, res) {
if (err) return done(err);
expect(res.body).to.be.an('array');
const items = res.body.filter(item => item.text === text);
expect(items).to.have.lengthOf.at.least(1);
done();
});
});
});
What Codex Got Right
- Framework detection: It detected that the app uses Express and set up supertest and chai correctly.
- Reasonable assumptions: It assumed a basic structure for the todo object, inferred the expected response, and validated the returned properties.
- Usable out of the box: The test ran successfully with minimal adjustments — we only had to ensure server.js exported the Express app properly.
Time saved: Writing this manually would take ~10 minutes, including setup. Codex delivered a runnable version in seconds.
Limitations & Caveats
Codex is powerful but has notable limitations:
- Occasional Hallucinations: May invent nonexistent functions or libraries
- Complexity Limitations: Struggles with large codebases or unconventional project structures
We also noticed that it doesn't yet adapt well to unconventional project structures or domain-specific tooling (e.g., internal SDKs or custom configuration patterns), unless explicitly described up front.
Verdict
Is Codex the future of software engineering? Yes—with an asterisk.
Codex significantly aids development but doesn't replace human creativity or intuition. It shifts developers' focus from coding to strategic thinking about code.
Ideal Users:
- Solo developers
- Teams automating routine tasks
- Educators and students
Codex amplifies developer intent, signaling a transformative era in software engineering.
Wrapping Up
Codex enables seamless collaboration across roles and accelerates development cycles. Whether you're new or experienced, Codex can meaningfully enhance your productivity.
Ready to integrate AI into your workflow? Start exploring Codex today.